矩形碰撞检测(不考虑旋转)
2d矩形碰撞检测,矩形平行于坐标轴
代码实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
|
let red = { x: 100, y: 100, wdith: 80, height: 60 }; let green = { x: 200, y: 200, wdith: 80, height: 60 };
let x = Math.abs(red.x - green.x) < red.width / 2 + green.width / 2; let y = Math.abs(red.y - green.y) < red.height / 2 + green.height / 2; if (x && y) { console.log('发生碰撞') };
|
放上思路图一张:
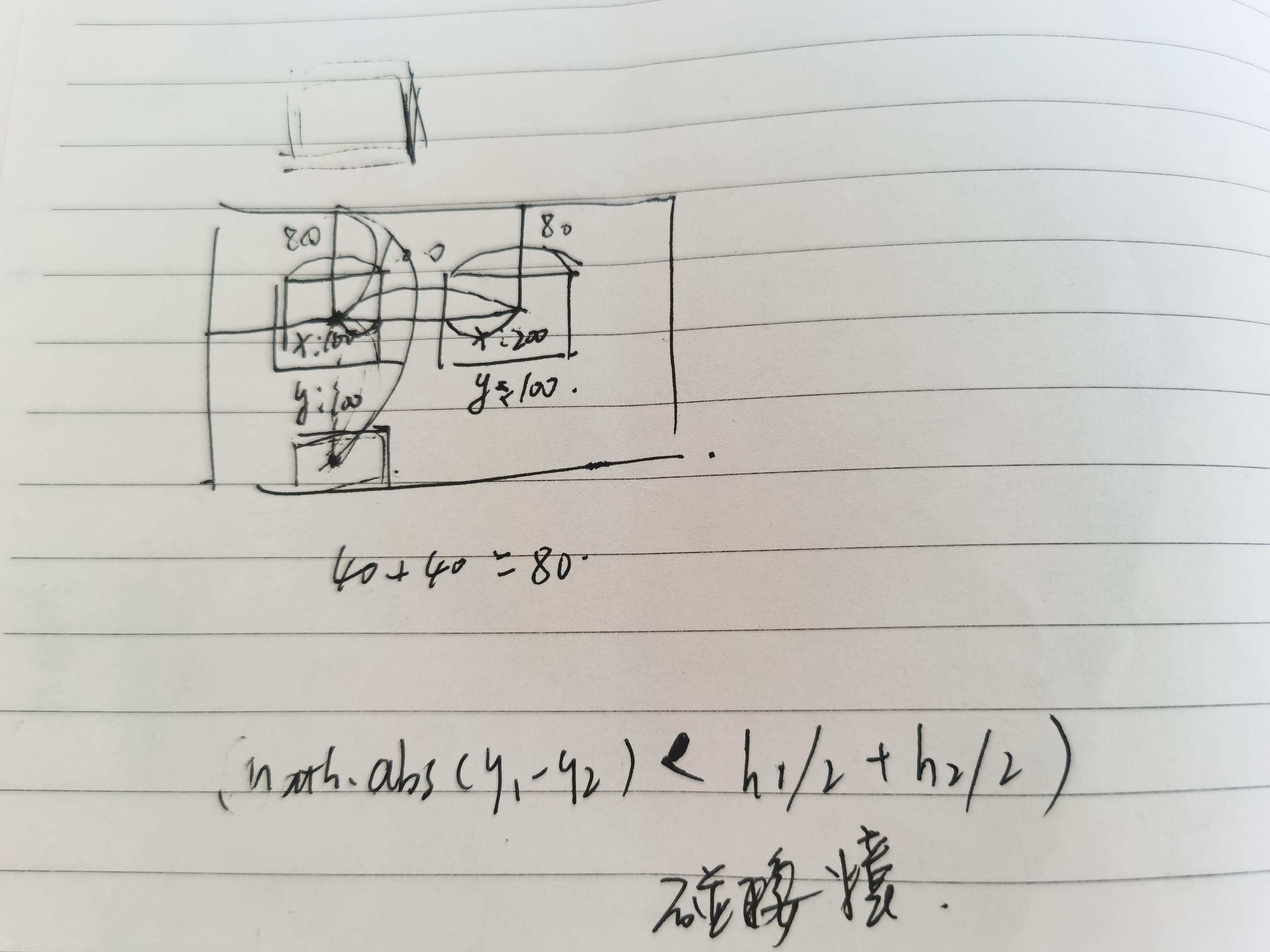
三种方法实现队列循环
题目要求:
实现红、绿、黄灯每隔指定时间不断重复交替亮灯。
第一种方法,利用promise链式调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| function red() { console.log('红灯') };
function green() { console.log('绿灯') };
function yellow() { console.log('黄灯') };
function light(cb, delay) { return new Promise((resolve, reject) => { setTimeout(() => { cb(); resolve(); }, delay) }) };
const step = function() { Promise.resolve().then(() => { return light(red, 3000) }).then(() => { return light(green, 2000) }).then(() => { return light(yellow, 1000) }).then(() => { step(); }) };
|
第二种方法,使用setInterval实现
1 2 3 4 5 6 7 8 9 10 11 12 13
| function light(l, t) { setTimeout(() => { console.log(l); }, t) }
function run() { light("绿灯", 3000); light("红灯", 2000); light("黄灯", 1000); }
setInterval(run, 6000);
|
第三种方法,使用async/awite
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| function light(delay) { return new Promise((resolve, reject) => { setTimeout(() => resolve(), delay) }) };
async function step(arr, times) { for (let i = 0; i < arr.length; i++) { console.log(arr[i]); await light(times[i]) } step(arr, times); };
step(['红', '绿', '黄'], [3000, 2000, 1000]);
|
文本溢出解决办法
问题:定宽溢出
解决方案:hover是弹出框提示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <div class='wrap'> <p>我是一个标题</p> <p>我是一个标题我是一个标题</p> <p>我是一个标题</p> <p>我是一个标题</p> <p>我是一个标题我是一个标题我是一个标题</p> </div>
.wrap { width: 150px; overflow: hidden; border: 1px solid red; }
p { white-space: nowrap; display: inline-block; }
p:hover { animation: move 2s infinite alternate; }
@keyframes move { 0% { transform: translate(0); } 100% { transform: translate(calc(-100% + 150px)); } }
|
vue路由守卫
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| router.beforeEach((to,from,next)=>{ if(to.matched.some(res=>res.meta.isLogin)){ if (sessionStorage['username']) { next(); }else{ next({ path:"/login", query:{ redirect:to.fullPath } }); }
}else{ next() } });
export default router;
|
to 表示将要跳转到的组件 (目标组件)
console.log(from); //(源组件)
next();
next 是一个函数
next() 进入下一个组件的钩子函数
next(false) 阻止跳转 中断导航
next(“/login”) 进入指定的组件的钩子函数